In a previous blog, I talked about using Alfresco to setup a simple Trivia Game and more specifically the Repository part of it, i.e., the meta-model and the structure I will use. In this second part, I will go through the REST-API commands that can be used to create new questions with their associated good/bad answers.
First of all, I would need to define the target endpoint and setup my credentials so that I can exchange with Alfresco. To keep things simple, I will use the Basic authentication with username/password. You could of course use a ticket or another authentication mechanism that is supported as you see fit. The “folder_id” is the “uuid” of the folder in which I prepared a few questions beginning of the year for my REST-API presentation and in which I will now add a new one for this blog:
$ base_url="https://alf-trivia.dbi-services.com"
$ endpoint="${base_url}/alfresco"
$ folder_id="e6395354-d38e-489b-b112-3549b521b04c"
$ username="admin"
$ read -s -p "Please enter the password of the '${username}' user for '${endpoint}': " password
Please enter the password of the 'admin' user for 'https://alf-trivia.dbi-services.com/alfresco':
$
$ auth=$(echo -n ${username}:${password} | base64)
$
I decided that the name of the folders inside Alfresco would be “QX” where X is the number of the question, from 1 to infinite. Therefore, to be able to create a new question, I would first need to find out how many are currently present in my quiz. For that purpose, I’m using the GET node’s children REST-API (listNodeChildren) that will list all the children of a specific node, here “${folder_id}“, with some of its details such as the children name, creation/modification date, creator/modifier, type, etc. In the result, all I would care about is the current total count of children that I can get easily using “JQ” (a JSON Processor command line utility):
$ response=$(curl -k -s -X GET "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${folder_id}/children" \
> -H "Authorization: Basic ${auth}" \
> -H "Accept: application/json")
$
$ echo ${response} | jq
{
"list": {
"pagination": {
"count": 6,
"hasMoreItems": false,
"totalItems": 6,
"skipCount": 0,
"maxItems": 100
},
"entries": [
{
"entry": {
"createdAt": "2023-01-18T10:42:14.957+0000",
"isFolder": true,
...
"name": "Q1",
"id": "d2afbdb6-2afb-4acc-85e7-61a800e96db3",
"nodeType": "cm:folder",
"parentId": "e6395354-d38e-489b-b112-3549b521b04c"
}
},
...
]
}
}
$
$ echo ${response} | jq -r ".list.pagination.totalItems"
6
$
Here, there are currently 6 children and therefore the next question to create would be “Q7“. For that purpose, I’m using the POST node’s children REST-API (createNode). The HTTP Method changes compared to the previous request and with the POST, it is possible to ask Alfresco to create a child instead of listing them. Here, I can directly assign the “dbi:dbi_trivia” aspect to it, so that it would enable the specific metadata for this node:
$ response=$(curl -k -s -X POST "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${folder_id}/children" \
> -H "Authorization: Basic ${auth}" \
> -H "Content-Type: application/json" \
> -H "Accept: application/json" \
> -d "{
> \"name\": \"Q${folder_nb}\",
> \"nodeType\": \"cm:folder\",
> \"aspectNames\": [
> \"dbi:dbi_trivia\"
> ],
> \"properties\": {
> \"cm:title\":\"Question ${folder_nb}\",
> \"cm:description\":\"Question #${folder_nb} of the TriviaGame\"
> }
> }")
$
$ echo ${response} | jq -r ".entry.id"
46d78e31-c796-4839-bac8-e6d5a7ff5973
$
With that, the new folder exists, and it has the associated aspect. The next step is purely optional, but I wanted to put a specific tag to all my questions, so that I can find them quicker in Share. For that purpose, I’m using the POST node’s tags REST-API (createTagForNode) on the newly create folder:
$ tag_name="triviaquestion"
$ response=$(curl -k -s -X POST "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${new_folder_id}/tags" \
> -H "Authorization: Basic ${auth}" \
> -H "Content-Type: application/json" \
> -H "Accept: application/json" \
> -d "{
> \"tag\": \"${tag_name}\"
> }")
$
The last step for the first script would then be to set the different aspect’s metadata into the newly create folder. For that purpose, I’m using the PUT node REST-API (updateNode), after prompting for the values to add exactly:
$ question="Is it possible to use Alfresco to play a Trivia Game?"
$ answer1="Obviously not, it's just an ECM!"
$ answer2="Only if using the Enterprise version..."
$ answer3="Of course, Alfresco can do everything"
$ answer4="I don't know"
$ correct_answer="Of course, Alfresco can do everything"
$
$ response=$(curl -k -s -X PUT "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${new_folder_id}" \
> -H "Authorization: Basic ${auth}" \
> -H "Content-Type: application/json" \
> -H "Accept: application/json" \
> -d "{
> \"properties\": {
> \"dbi:dbi_question\":\"${question}\",
> \"dbi:dbi_answer1\":\"${answer1}\",
> \"dbi:dbi_answer2\":\"${answer2}\",
> \"dbi:dbi_answer3\":\"${answer3}\",
> \"dbi:dbi_answer4\":\"${answer4}\",
> \"dbi:dbi_correct_answer\":\"${correct_answer}\"
> }
> }")
$
$ echo ${response} | jq
{
"entry": {
"aspectNames": [
"cm:titled",
"cm:auditable",
"dbi:dbi_trivia",
"cm:taggable"
],
"createdAt": "2023-11-03T10:12:49.804+0000",
"isFolder": true,
...
"name": "Q7",
"id": "46d78e31-c796-4839-bac8-e6d5a7ff5973",
"nodeType": "cm:folder",
"properties": {
"cm:title": "Question 7",
"dbi:dbi_question": "Is it possible to use Alfresco to play a Trivia Game?",
"dbi:dbi_correct_answer": "Of course, Alfresco can do everything",
"dbi:dbi_answer4": "I don't know",
"dbi:dbi_answer3": "Of course, Alfresco can do everything",
"dbi:dbi_answer2": "Only if using the Enterprise version...",
"dbi:dbi_answer1": "Obviously not, it's just an ECM!",
"cm:description": "Question #7 of the TriviaGame",
"cm:taggable": [
"6017bd2f-05d2-4828-9a1d-a418cf43a84e"
]
},
"parentId": "e6395354-d38e-489b-b112-3549b521b04c"
}
}
$
Putting everything together into a small bash script and then executing it again to make sure everything works, to create the 8th question:
$ cat triviaAdd.sh
#!/bin/bash
# Define endpoint, credentials, and folder ID
base_url="https://alf-trivia.dbi-services.com"
endpoint="${base_url}/alfresco"
share="${base_url}/share/page"
folder_id="e6395354-d38e-489b-b112-3549b521b04c"
username="admin"
read -s -p "Please enter the password of the '${username}' user for '${endpoint}': " password
auth=$(echo -n ${username}:${password} | base64)
tag_name="triviaquestion"
# Get number of children
echo
echo
echo "Fetching the current number of questions from Alfresco..."
echo
response=$(curl -k -s -X GET "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${folder_id}/children" \
-H "Authorization: Basic ${auth}" \
-H "Accept: application/json")
num_children=$(echo ${response} | jq -r ".list.pagination.totalItems")
folder_nb="$((num_children+1))"
# Create new folder
response=$(curl -k -s -X POST "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${folder_id}/children" \
-H "Authorization: Basic ${auth}" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d "{
\"name\": \"Q${folder_nb}\",
\"nodeType\": \"cm:folder\",
\"aspectNames\": [
\"dbi:dbi_trivia\"
],
\"properties\": {
\"cm:title\":\"Question ${folder_nb}\",
\"cm:description\":\"Question #${folder_nb} of the TriviaGame\"
}
}")
new_folder_id=$(echo ${response} | jq -r ".entry.id")
echo -e "\033[32;1m --> A new folder 'Q${folder_nb}' has been created: ${share}/folder-details?nodeRef=workspace://SpacesStore/${new_folder_id}\033[0m"
echo
# Add the tag
response=$(curl -k -s -X POST "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${new_folder_id}/tags" \
-H "Authorization: Basic ${auth}" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d "{
\"tag\": \"${tag_name}\"
}")
echo -e "\033[32;1m --> The tag '${tag_name}' has been added to the folder\033[0m"
echo
# Add question, answers and correct answer
read -p "Enter the question: " question
read -p "Enter the answer #1: " answer1
read -p "Enter the answer #2: " answer2
read -p "Enter the answer #3: " answer3
read -p "Enter the answer #4: " answer4
read -p "Enter the correct answer: " correct_answer
response=$(curl -k -s -X PUT "${endpoint}/api/-default-/public/alfresco/versions/1/nodes/${new_folder_id}" \
-H "Authorization: Basic ${auth}" \
-H "Content-Type: application/json" \
-H "Accept: application/json" \
-d "{
\"properties\": {
\"dbi:dbi_question\":\"${question}\",
\"dbi:dbi_answer1\":\"${answer1}\",
\"dbi:dbi_answer2\":\"${answer2}\",
\"dbi:dbi_answer3\":\"${answer3}\",
\"dbi:dbi_answer4\":\"${answer4}\",
\"dbi:dbi_correct_answer\":\"${correct_answer}\"
}
}")
echo -e "\033[32;1m --> The question and answers have been added to the folder\033[0m"
echo
$
$
$ # Execute the script to add a question
$ ./triviaAdd.sh
Please enter the password of the 'admin' user for 'https://alf-trivia.dbi-services.com/alfresco':
Fetching the current number of questions from Alfresco...
--> A new folder 'Q8' has been created: https://alf-trivia.dbi-services.com/share/page/folder-details?nodeRef=workspace://SpacesStore/0b147c71-70fd-498e-9bf6-d40a738699fa
--> The tag 'triviaquestion' has been added to the folder
Enter the question: Is this working as it should?
Enter the answer #1: Of course...
Enter the answer #2: Maybe?
Enter the answer #3: Definitively not
Enter the answer #4: Why do you ask me???
Enter the correct answer: Of course...
--> The question and answers have been added to the folder
$
Unfortunately I cannot display the colors in our blog platform for the code sections, but if you look at the source code, you will see the script has that so that the output is clearer. Looking into Alfresco Share shows the newly created folder with the proper metadata:
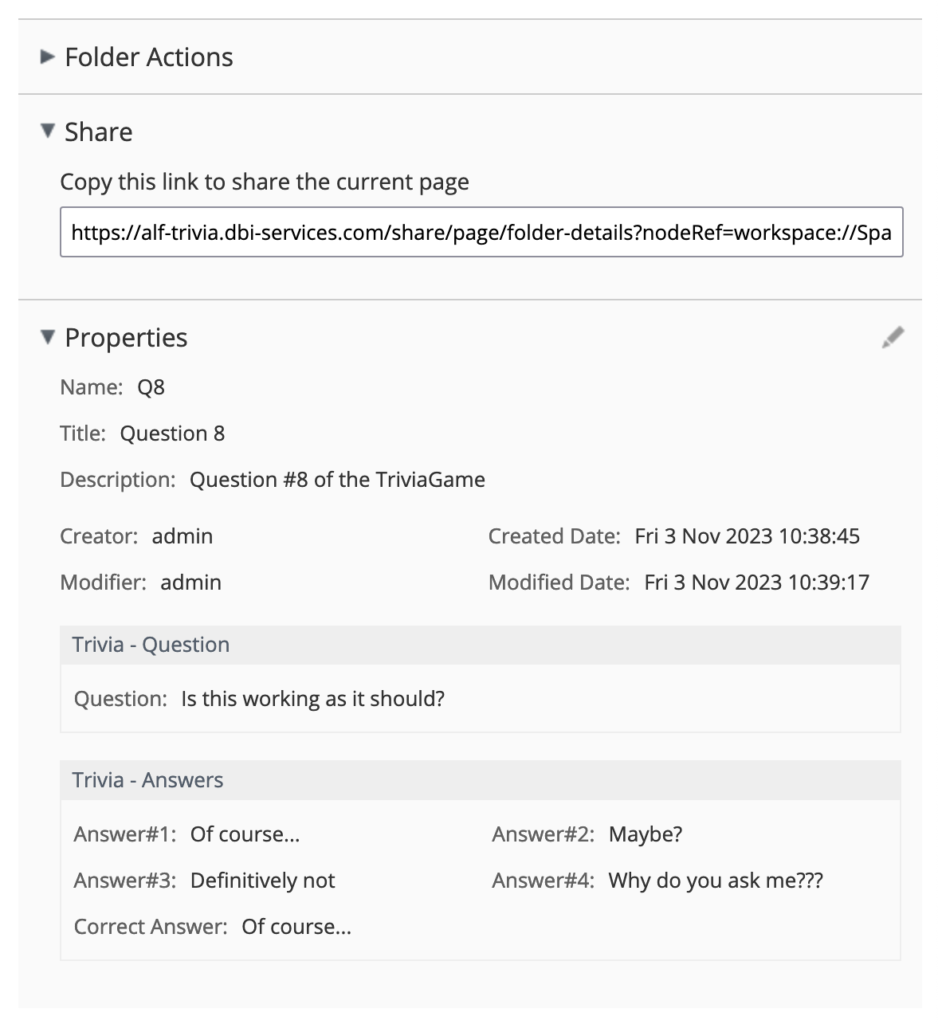
That concludes the second part of this blog. If you are familiar with REST-API, writing this kind of small script shouldn’t take you more than a few minutes, let’s say 30 minutes if you want to add colors as I did and wrapper/information around, to have something that can be presented. The first part of this blog is available here and the third part of this blog here.