Introduction
WebHooks are HTTP callbacks that are triggered by events. In case of GitLab these can be events like pushing some code, or creating an issue, or pushing some comments.
In this quick post I will explain how to add a WebHook to a project and how to execute some external code when a Gitlab event is triggered.
VM preparation
Do not forget to open the firewall port for TCP
protocol, on 8200
port must be opened.
[root@wb ~]# firewall-cmd --list-all
public (active)
target: default
icmp-block-inversion: no
interfaces: ens3
sources:
services: dhcpv6-client http ssh
ports:
protocols:
forward: no
masquerade: no
forward-ports:
source-ports:
icmp-blocks:
rich rules:
[root@wb ~]# firewall-cmd --zone=public --add-port=8200/tcp
success
[root@wb ~]# firewall-cmd --list-all
public (active)
target: default
icmp-block-inversion: no
interfaces: ens3
sources:
services: dhcpv6-client http ssh
ports: 8200/tcp
protocols:
forward: no
masquerade: no
forward-ports:
source-ports:
icmp-blocks:
rich rules:
Python code
An easy way is to use Flask
, to retrieve the HTTP
frame.
# app.py
from flask import Flask, request, abort
import json
app = Flask(__name__)
@app.route('/my_webhook', methods=['POST'])
def my_webhook():
if request.method == 'POST':
print(json.dumps(request.json, indent=2))
return 'success', 200
else:
abort(400)
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8200, debug=True)
- The
Flask
will launch a http server which is listen on all interfaces (0.0.0.0
) en on port8200
- The path to be used in the
HTTP POST
call ismy_webhook
- Also I used the debug mode to get the most traces.
- The
Flask
application is waiting aPOST
request (line 10). And answersuccess
. if the POST request is received. - Otherwise, return
400
HTTP code.
Start the Flask application
(venv) [opc@wb ~]$ python app.py
* Serving Flask app 'app' (lazy loading)
* Environment: production
WARNING: This is a development server. Do not use it in a production deployment.
Use a production WSGI server instead.
* Debug mode: on
* Running on all addresses.
WARNING: This is a development server. Do not use it in a production deployment.
* Running on http://172.168.0.40:8200/ (Press CTRL+C to quit)
* Restarting with stat
* Debugger is active!
* Debugger PIN: 295-390-357
Define the GitLab WebHook
You must have the role maitainer
on your project, in order to define WebHooks
Select Settings -> WebHooks from the left GitLab Menu
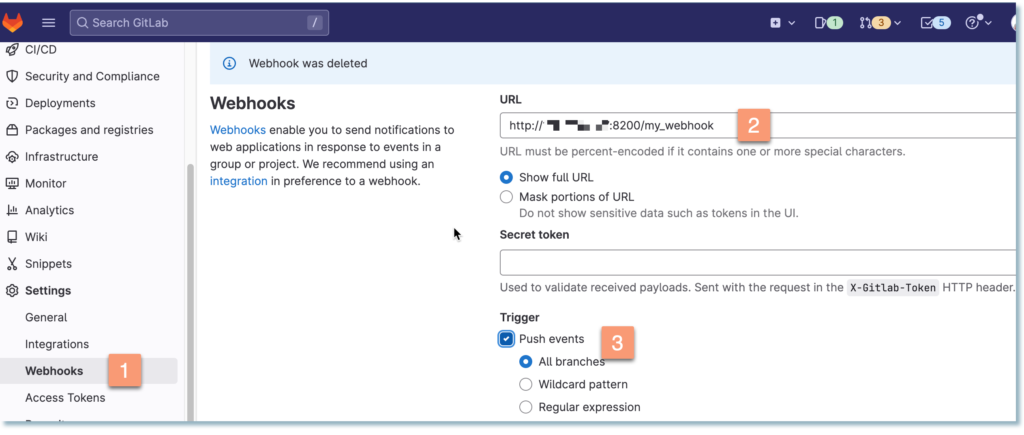
Once the WebHook was saved it can be tested by pressing the Test button. I choose to test a Push event which is triggered at each push is issued from Git.
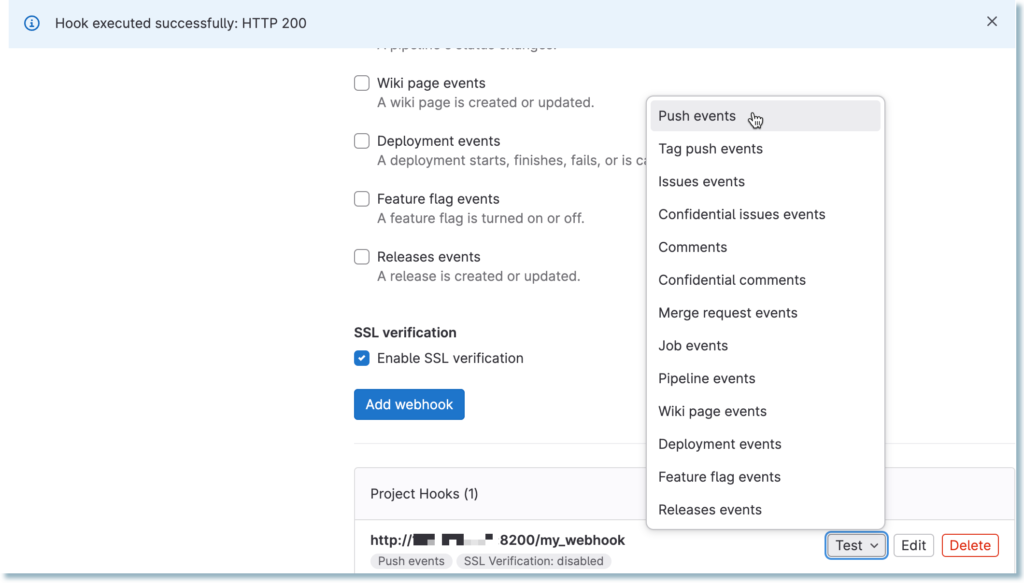
On the VM terminal we get the json trace of the POST
request:
..........
* Debugger is active!
* Debugger PIN: 295-390-357
{
"object_kind": "push", =====> Event type PUSH
"event_name": "push",
"before": "6aa30335b09*****53183fb28bb84aada5cf",
"after": "a48e187ef217a******62498378e5023d355",
"ref": "refs/heads/main",
"checkout_sha": "a48e187ef21********9490562498378e5023d355",
"message": null,
"user_id": 1177**25,
"user_name": "your username ",
"user_username": "your.username",
"user_email": "",
"user_avatar": "https://gitlab.com/uploads/-/system/user/avatar/****/avatar.png",
"project_id": 3887**93,
"project": {
"id": 38878**3,
.....
Conclusion
The use of WebHooks from GitLab to run external code represents another facility in DevOps tools.
Their implementation is easy and immediate. An typical example of use case is the integration with Ansible Automation Controller, to run Ansible jobs.
References
https://docs.gitlab.com/ee/user/project/integrations/webhooks.html