Following on from my previous article: Create REST API from your database in minute with Feathers.js, today I want to add a UI to explore the Feathers.js API.
When we provide an API, it’s common to make a tool available to explore the exposed methods.
Swagger UI is one such tool, and fortunately, in the Feathers.js ecosystem, a package is available for this purpose.
First step: install and declare the package
In my previous article, I created a Feathers.js project using Koa as HTTP framework, TypeScript as language and NPM as package manager. To install the feathers-swagger package, I use the command :
npm install feathers-swagger swagger-ui-dist koa-mount koa-static
Once I’ve installed the necessary packages, I just need to declare the Swagger interface in my project.
At the beginning of the src/app.ts file, I add the swagger package import:
import swagger from 'feathers-swagger';
Then, before the “app.configure(services)”, I add the Swagger declaration:
app.configure(swagger({
specs: {
info: {
title: 'Workshop API ',
description: 'Workshop API rest services',
version: '1.0.0',
}
},
ui: swagger.swaggerUI({ docsPath: '/docs' }),
}))
Note: the position of the declaration in the file is very important: if it’s after the services configuration, the Swagger interface will be empty.
Second step: expose the service in Swagger UI
Once the feathers-swagger package has been installed and initialized in the project, all I have to do is tell the services what to expose. To do this, I add a docs part to my service workshop service in the file (src/services/workshop/workshop.ts).
// A configure function that registers the service and its hooks via `app.configure`
export const workshop = (app: Application) => {
// Register our service on the Feathers application
app.use(workshopPath, new WorkshopService(getOptions(app)), {
// A list of all methods this service exposes externally
methods: workshopMethods,
// You can add additional custom events to be sent to clients here
events: [],
docs: createSwaggerServiceOptions({
schemas: { workshopSchema, workshopDataSchema, workshopPatchSchema, workshopQuerySchema },
docs: {
// any options for service.docs can be added here
description: 'Workshop service',
}
}),
})
Don’t forget to add the necessary imports at the beginning of the file:
import {
workshopDataValidator,
workshopPatchValidator,
workshopQueryValidator,
workshopResolver,
workshopExternalResolver,
workshopDataResolver,
workshopPatchResolver,
workshopQueryResolver,
workshopDataSchema,
workshopPatchSchema,
workshopQuerySchema,
workshopSchema
} from './workshop.schema'
import type { Application } from '../../declarations'
import { WorkshopService, getOptions } from './workshop.class'
import { workshopPath, workshopMethods } from './workshop.shared'
import { createSwaggerServiceOptions } from 'feathers-swagger'
Important : in the case of a TypeScript project, the docs property is not recognized. An additional type declaration must therefore be added. In the src/declarations.ts file, add :
declare module '@feathersjs/feathers' {
interface ServiceOptions {
docs?: ServiceSwaggerOptions;
}
}
Testing the UI
As usual, to test the service, I use the command :
npm run dev
Once the server has been started, simply access the docs page: http://localhost:3030/docs/
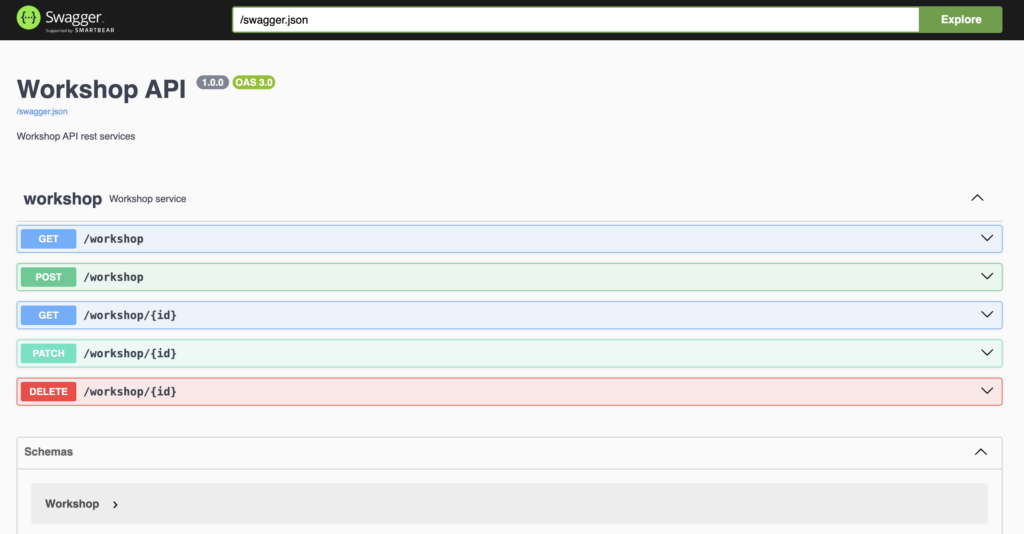
From the Swagger interface, I can test API methods:
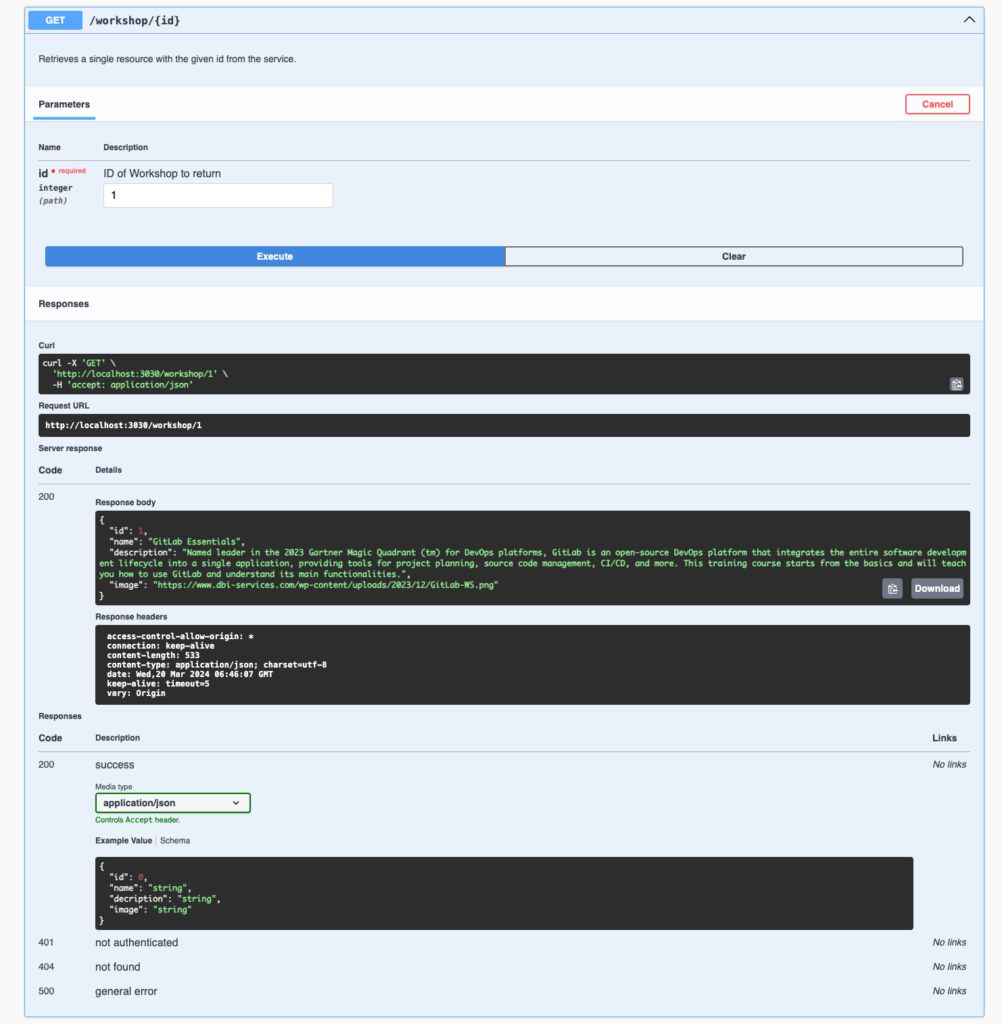
Conclusion
Adding a UI to explore the Feathers.js API is quick and easy, thanks to our ecosystem packages.
Swagger UI is a very useful tool, and adding it to your project is a good idea in many cases, such as :
- When developing the API itself, to test methods and make sure they work properly
- For the front-end developer using the API, it’s easy to know which methods are available and how to use them.
- In the case of a public API, users can easily support and test methods using the UI